404. Sum of Left Leaves
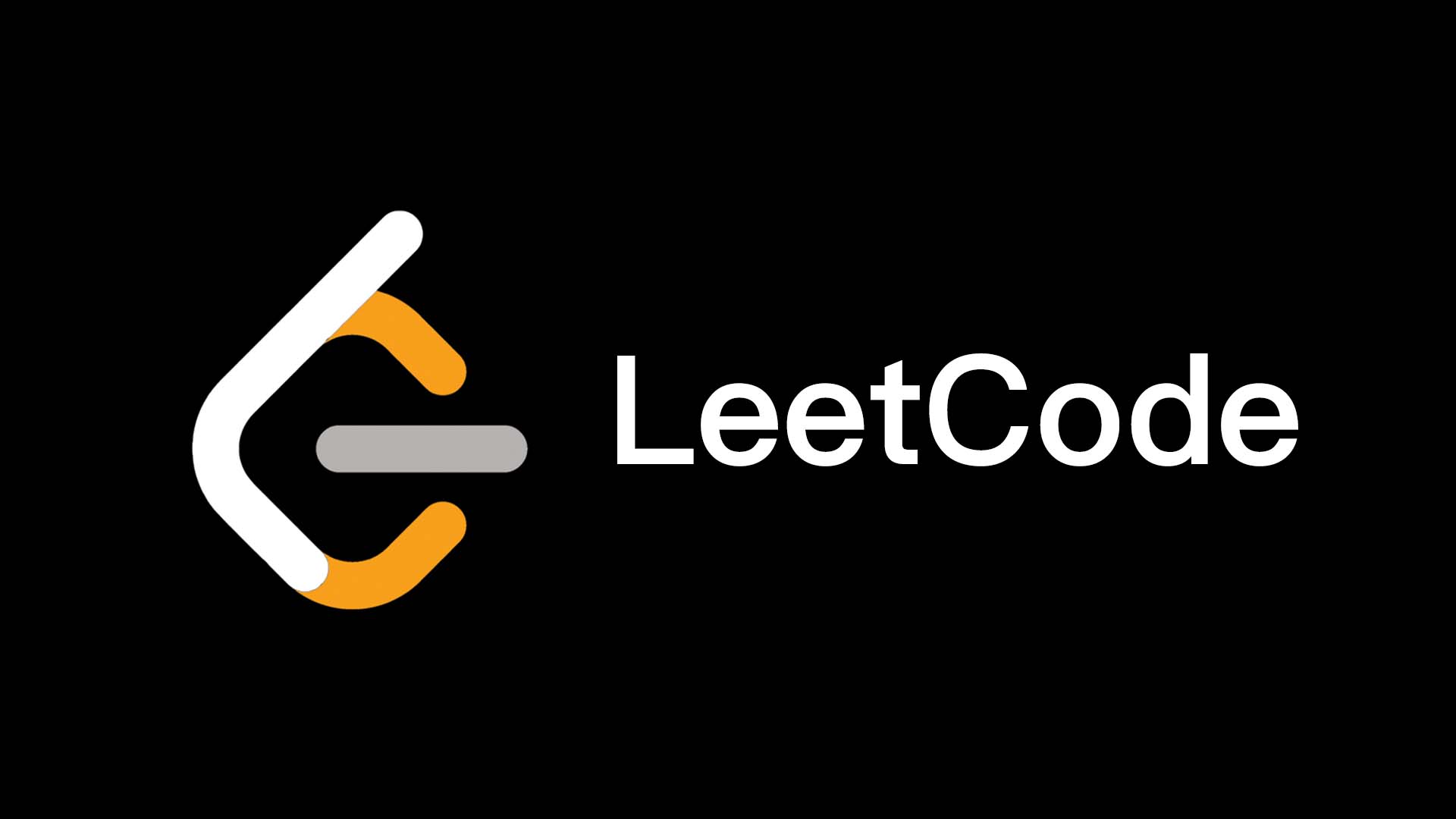
Overview
We need to find the sum of the left leaves of a binary tree.
The left leaves cannot have any child nodes, including left child nodes and right child nodes.
We will separately use DFS and BFS to resolve this problem.
Solution 1: Depth-first search (DFS)
Algorithm
Make sumOfLeftLeaves
a recursive function.
-
If the
root
node is None, return 0. -
If the
left
node of theroot
node is not None
and theleft
node of theleft
node of theroot
node is None
and theright
node of theleft
node of theroot
node is None:- Return the
value
of theleft
node of theroot
node
plussumOfLeftLeaves
with theright
node of theroot
node.
We only find the sum of the left nodes which don't have child nodes.
- Return the
-
Return
sumOfLeftLeaves
with theleft
node of theroot
node plussumOfLeftLeaves
with theright
node of theroot
node.\We'll recursively start from the root node to the deepest node.
Implement
class Solution: def sumOfLeftLeaves(self, root: Optional[TreeNode]) -> int: if root is None: return 0 if root.left and root.left.left is None and root.left.right is None: return root.left.val + self.sumOfLeftLeaves(root.right) return self.sumOfLeftLeaves(root.left) + \ self.sumOfLeftLeaves(root.right)
Complexity Analysis
Time complexity: O(n)
We traverse all nodes of the binary tree, which takes O(n) time.
Space complexity: O(n)
The recursive function uses memory to store the function state, which takes O(n) space.
Solution 2: Breadth-first search (BFS)
Algorithm
Without using a recursive function, we use queue instead.
-
Initialize
total
to 0 to store the sum of the left nodes' values.
Initializequeue
to store the nodes at the same depth that we'll traverse. -
If
queue
is not empty:-
Pop the leftmost node of
queue
asnode
. -
If the
left
node of thenode
is not None
and theleft
node of theleft
node of thenode
is None
and theright
node of theleft
node of thenode
is None:- Increase
total
by thevalue
of theleft
node of thenode
.
- Increase
-
If the
left
node of thenode
is not None:- Append the
left
node of thenode
toqueue
for the next traversal.
- Append the
-
If the
right
node of thenode
is not None:- Append the
right
node of thenode
toqueue
for the next traversal.
- Append the
-
-
Return
total
as the sum of the left nodes which don't have child nodes.
Implement
class Solution: def sumOfLeftLeaves(self, root: Optional[TreeNode]) -> int: total = 0 queue = deque([root]) while queue: node = queue.popleft() if node.left and node.left.left is None and node.left.right is None: total += node.left.val if node.left is not None: queue.append(node.left) if node.right is not None: queue.append(node.right) return total
Complexity Analysis
Time complexity: O(n)
We traverse all nodes of the binary tree, which takes O(n) time.
Space complexity: O(1)
The queue at most takes the maximum number of nodes at the same depth,
which are always fewer than the total number of nodes in the binary tree.